By Jesse Schutt
How We Keep Code Clean at Zaengle
Let's be honest, we all like systematic, uniform code.
However, maintaining a respectable level of cleanliness across multiple developers' systems can be a challenge. Thankfully there are a few tools to help unify our editors so that our code styling gets along!
ESlint
ESLint enforces rules across our javascript files and is configurable via simple declarations.
Install the required packages:
yarn add eslint eslint-plugin-vue babel-eslint --dev
It's likely the each project will have unique linting needs, but we've had good luck using this as a starting point. Create a file at the root of your project called .eslintrc.json
with the following code:
{
"extends": [
"plugin:vue/recommended",
"eslint:recommended"
],
"rules": {
"no-console": 1,
"indent": [
"error",
2
]
},
"parserOptions": {
"parser": "babel-eslint"
}
}
PHPStorm should automatically pick up the .eslint configuration and begin displaying warnings/errors. If it does not, make sure ESLint is enabled in your PHPStorm preferences.
.editorconfig
EditorConfig is a standard to share generic settings across multiple editors. Check here to see if your preferred editor supports the .editorconfig file.
Things like tab/spaces, indentation, and line-break preferences are specified in a single document called .editorconfig
which is placed in the root of your project.
[*]
charset=utf-8
end_of_line=lf
insert_final_newline=false
indent_style=space
indent_size=4
[{*.sht,*.html,*.shtm,*.shtml,*.ng,*.htm}]
indent_style=space
indent_size=2
[{composer.lock,.eslintrc,.prettierrc,.babelrc,jest.config,.stylelintrc,*.bowerrc,*.jsb3,*.jsb2,*.json,*.graphqlconfig}]
indent_style=space
indent_size=2
[*.csv]
indent_style=tab
tab_width=1
[*.scss]
indent_style=space
indent_size=2
[{*.cjs,*.js}]
indent_style=space
indent_size=2
[{tsconfig.lib.json,tsconfig.app.json,tsconfig.e2e.json,tsconfig.spec.json,tsconfig.json}]
indent_style=space
indent_size=2
[*.js.map]
indent_style=space
indent_size=2
[*.vue]
indent_style=space
indent_size=2
[{*.yml,*.yaml}]
indent_style=space
indent_size=2
Setting Up PHPStorm
Most of the developers at Zaengle use PHPStorm so these instructions are specific to that platform. (You can still use ESLint and EditorConfig in other editors but you are on your own to figure them out!)
- Check that the snippet above is in a
.editorconfig
file at the root of your project. - Search the plugin repository for "editorconfig" and install the plugin titled "EditorConfig".
- Visit
Preferences / Code Style
and confirmEnable EditorConfig support
is checked.
Your code style settings should now use the team-wide defaults laid out in the config file!
In addition to the .editorconfig file you should configure the following items manually:
Gotcha: Managing Default Block Indentation
The plugin:vue-recommended
rules require that javascript not be indented within a script
tag and PHPStorm indents it by default. Thankfully we can override which items should not be indented like this:
- Visit
Preferences / Code Style / HTML / Other
and look for theDo not indent children of:
setting. - Remove
thead
,tbody
, andtfoot
. - Add
script
. - Save your change
Applying Formatting
There are two ways to apply formatting to your code in PHPStorm:
Method #1 - Press cmd + option + l
to apply the .editorconfig formatting and specific block indentation.
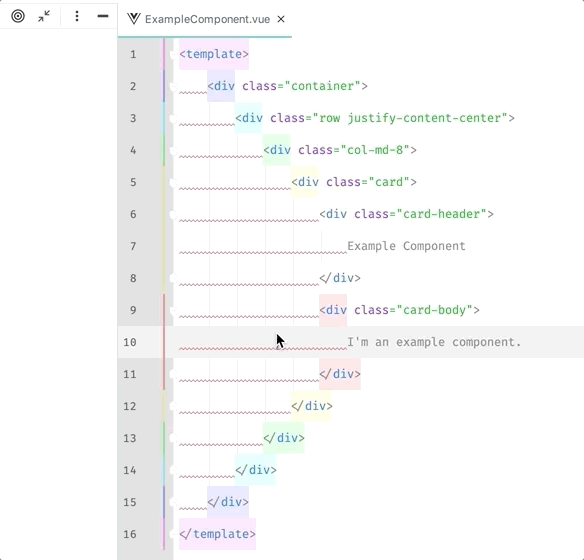
Method #2 - Place your cursor over a section that has an error/warning highlight and press option + return
to bring up a dialog. Select ESLint: fix current file
to apply the styles specified in the .eslintrc.json file.
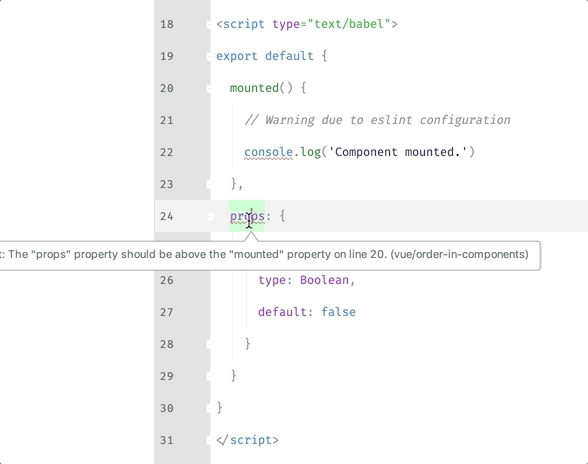
Conclusion
With a few simple tools and customizations to our editors we've come a long way in code uniformity!
Want to read more tips and insights on working with a website development team that wants to help your organization grow for good? Sign up for our bimonthly newsletter.
By Jesse Schutt
Director of Engineering
Jesse is our resident woodworker. His signature is to find the deeper meaning in a project and the right tool for the job.